The Mixmax REST API allows you programmatic access to read and modify your data in Mixmax. It's available to all users. API access is restricted to Mixmax annual plans of Growth+ or Enterprise. APIs are available over HTTPS/TLS only.
If you'd like a trial account to use as a developer sandbox, please email us at [email protected] with details about what you're building.
Please let us know how you're using the Mixmax API and what we can do to make it better. Email us at [email protected].
Authentication
To begin, create a developer token in your Mixmax Settings ▸ Integrations.
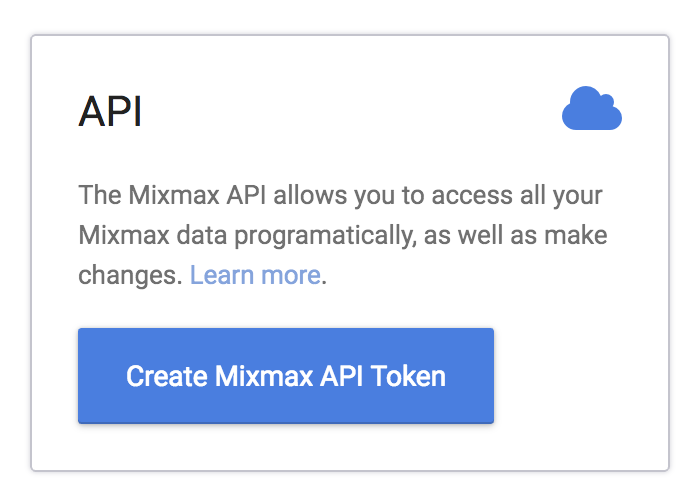
Keep your token in a safe place, as it will only be given to you once and then stored securely. You can use your token to call the API by passing it as the value of the apiToken
querystring parameter. or using the HTTP custom header X-API-Token
. Here's an example of the latter using curl:
curl --header "X-API-Token: 45e99c6b-386d-4aa1-a2b4-296568a40ff2" https://api.mixmax.com/v1/users/me
Or in the browser, using jQuery:
$.ajax({
url: 'https://api.mixmax.com/v1/users/me',
headers: {
'X-API-Token': '45e99c6b-386d-4aa1-a2b4-296568a40ff2'
}
});
You can also use your API token directly in this documentation. Underneath the API you want to use, click the user authentication icon next to the "Try It" button:
and paste your token in, like this:
Then you can use any API and see the live results.
Request content types
The API accepts POST, PATCH, and PUT request bodies to be passed in the following content-type formats: application/x-www-form-urlencoded
or application/json
.
Pagination
API responses that return collections are always returned with the newest object first (by when they were created). When querying a collection through the API, the following querystrings are supported:
limit
- {Number} The number of results you'd like to get back in each page. Defaults to 50. The upper limit is usually 300, but might be higher for some APIs.next
- {String} The string "cursor" to start fetching the next page of results (from a previous API response - see below).previous
- {String} The string "cursor" to start fetching the previous page of results if you're walking pages backwards (from a previous API response - see below).
All successful (HTTP status code: 200) responses for collections will have the following structure:
results
{Array} An array of objects that are returned by the query.next
- {String} A cursor used to fetch the next page in the collection, for pagination. The next page can be fetched by passing?next=<value>
on the querystring of the next request.hasNext
- {Boolean} Set to true if querying withnext
will return more results. If this is set tofalse
you can still query the next page to pick up results that were added after the first query was run.previous
- {String} A cursor used to fetch the previous page of the collection, for pagination. The previous page can be fetched by passing?previous=<value>
on the querystring of the next request.hasPrevious
- {Boolean} Set to true if querying withprevious
will return more results. If this is set tofalse
you can still query the previous page to pick up results that were added after this query was originally run. Since most APIs are reverse-chronologically ordered, you can poll?previous=<value>
to pick up new objects that are added to a collection.
Example:
API polls?limit=10
returns the response:
{
results: [ ... ],
next: "MTQ3NzQ0ODc1MTg4Mg",
hasNext: true
}
To query the next page, pass the next
parameter on the URL: https://api.mixmax.com/v1/polls?limit=10&next=MTQ3NzQ0ODc1MTg4Mg. The next
and previous
parameters are always URL safe strings so they don't need to be escaped.
You can learn more about our approach to API pagination on our Engineering Blog: API Paging Built the Right Way.
Fields & expansions
By default, all APIs return all properties of the response objects. For APIs that return collections, you can have it return only specific fields by passing a comma-delimited list of field names (using dot notation for nested properties) on the querystring as fields
. For example: https://api.mixmax.com/v1/qa?fields=_id,title,users.userId will return just the _id
, title
, and the userId
property on the nested user
object.
Some APIs support expansions that will return more detail about an existing property (usually a linked resource) OR a new meta-property (e.g. count
of the number of subresources). They are documented in individual API documentation where they are supported (under Query Params). Expansions are requested by passing a comma-delimited list of field names on the querystring as expand=
, e.g. https://api.mixmax.com/v1/sequences/1234?expand=stages,userId.
Rate limiting
API requests are limited to 120 requests per a 60 second window (per IP address and user). If you exceed your quota you will receive a HTTP 429
response with a Retry-After
HTTP header.
Some APIs have stricter rate limiting that is noted in the documentation for that API.
Every response from the API contains the following HTTP headers to let you know where you are at in your rate limiting:
HTTP Header | |
---|---|
X-RateLimit-Limit | Total number of requests allowed in this time window |
X-RateLimit-Reset | Timestamp in UTC epoch seconds when rate limiting resets |
X-RateLimit-Remaining | Number of requests remaining for this time window |
Errors
The Mixmax API uses standard HTTP response codes to indicate errors. Codes in the 2xx range indicate success, codes in the 4xx range indicate an error that failed given the information provided (e.g., a required parameter was omitted or document doesn't exist), and codes in the 5xx range indicate an error with Mixmax servers (these are rare). If there is a reason for the error, it will be returned in the JSON payload under the key message
, e.g.
{
message: "Missing parameter teamIds"
}